Features > Google Authentication
Implementing Google Authentication in your mobile app is the fastest way of collecting user data with the opportunity of less likely to lose user engagement in your mobile application. It is because signing in by gmail is the easiest and fastest way for mobile app users most of the time and they less likely churn because they are asked to login with their Google account. 📊
Setup Google Authentication
1. Go to the Firebase console
2. Click on Authentication page and click on Sign-in Method Tab.
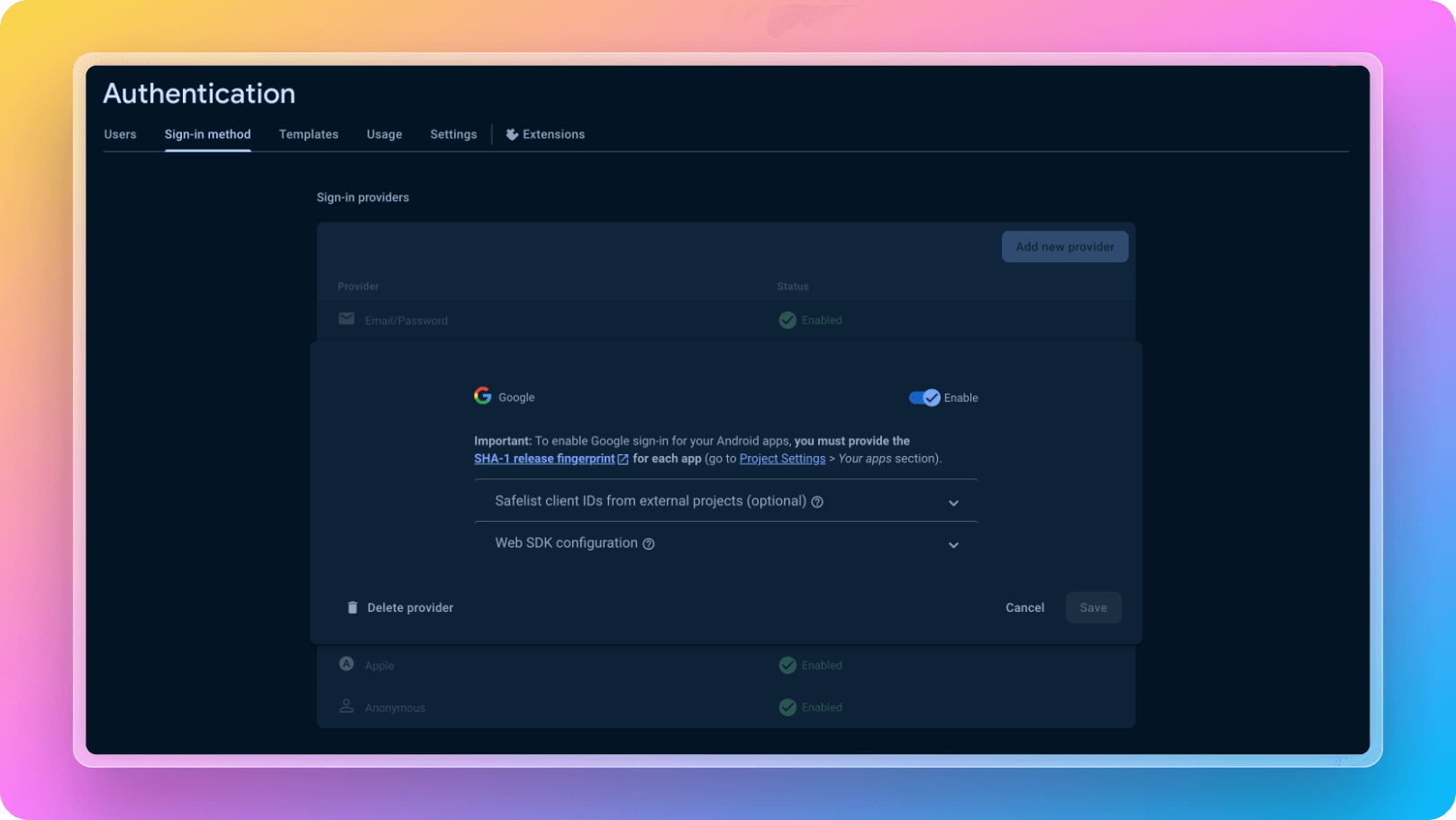
3. Enable Google authentication as seen on the screenshot above.
4. (Android Only) For Google Authentication to work on Android devices, we need to enter SHA1 or SHA256 fingerprint to the our already created Android app. (This is explained in Build in 5 Minutes Docs) After you go to react-native-starter-ai-mobile/android folder, SHA256 or SHA1 fingerprints can easily be checked on your computer by running the commands depending on your OS accordingly:
MacOS:
1keytool -list -v -keystore ~/.android/debug.keystore -alias androiddebugkey -storepass android -keypass android
Windows
1keytool -list -v -keystore ".androiddebug.keystore" -alias androiddebugkey -storepass android -keypass android
5. After you run one of the commands above, you will get something like below:
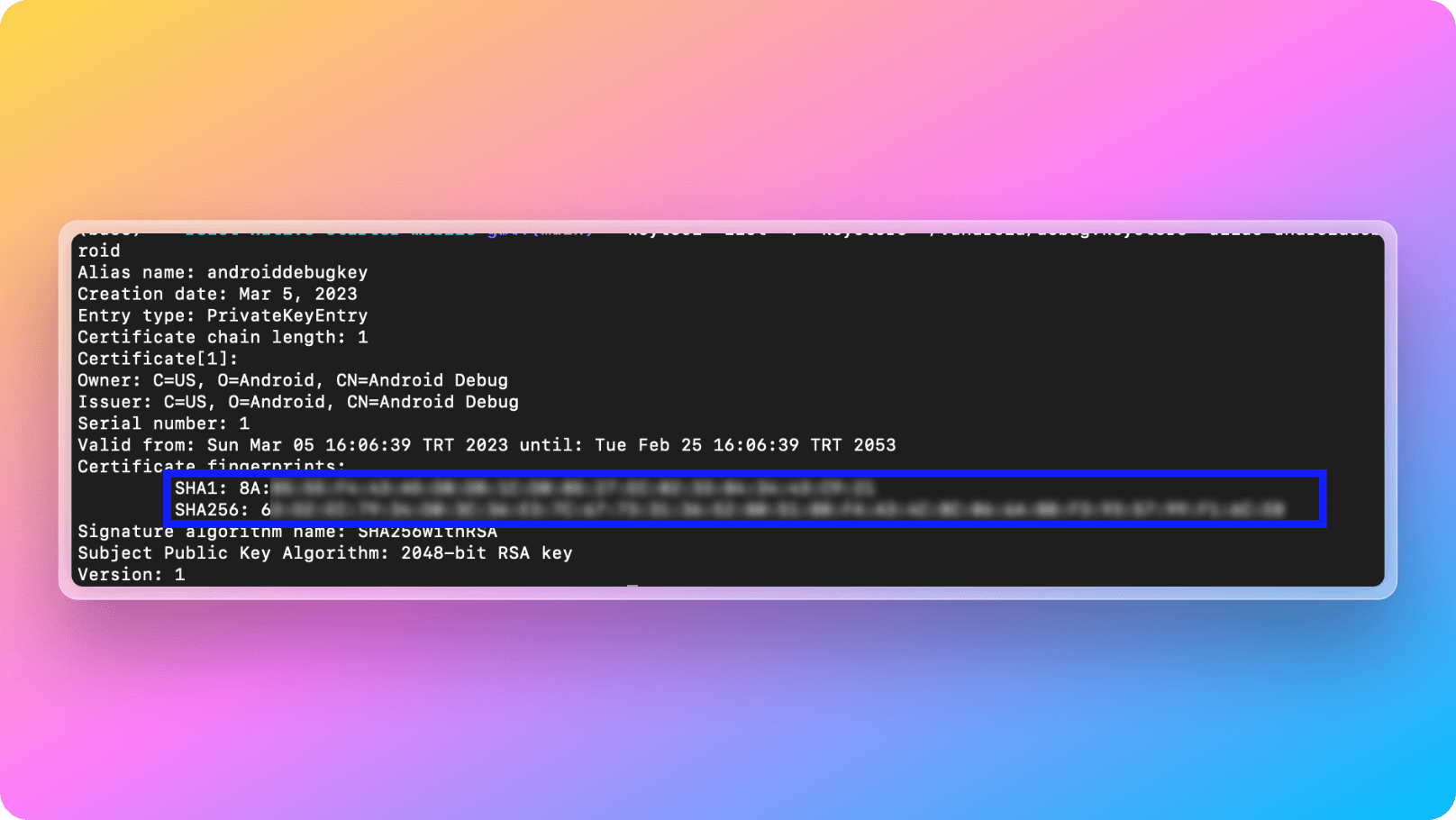
6. You need to copy one of them(SHA1 or SHA256) and paste it into your Firebase Android App project in the Project Settings Page like in the screenshot below:
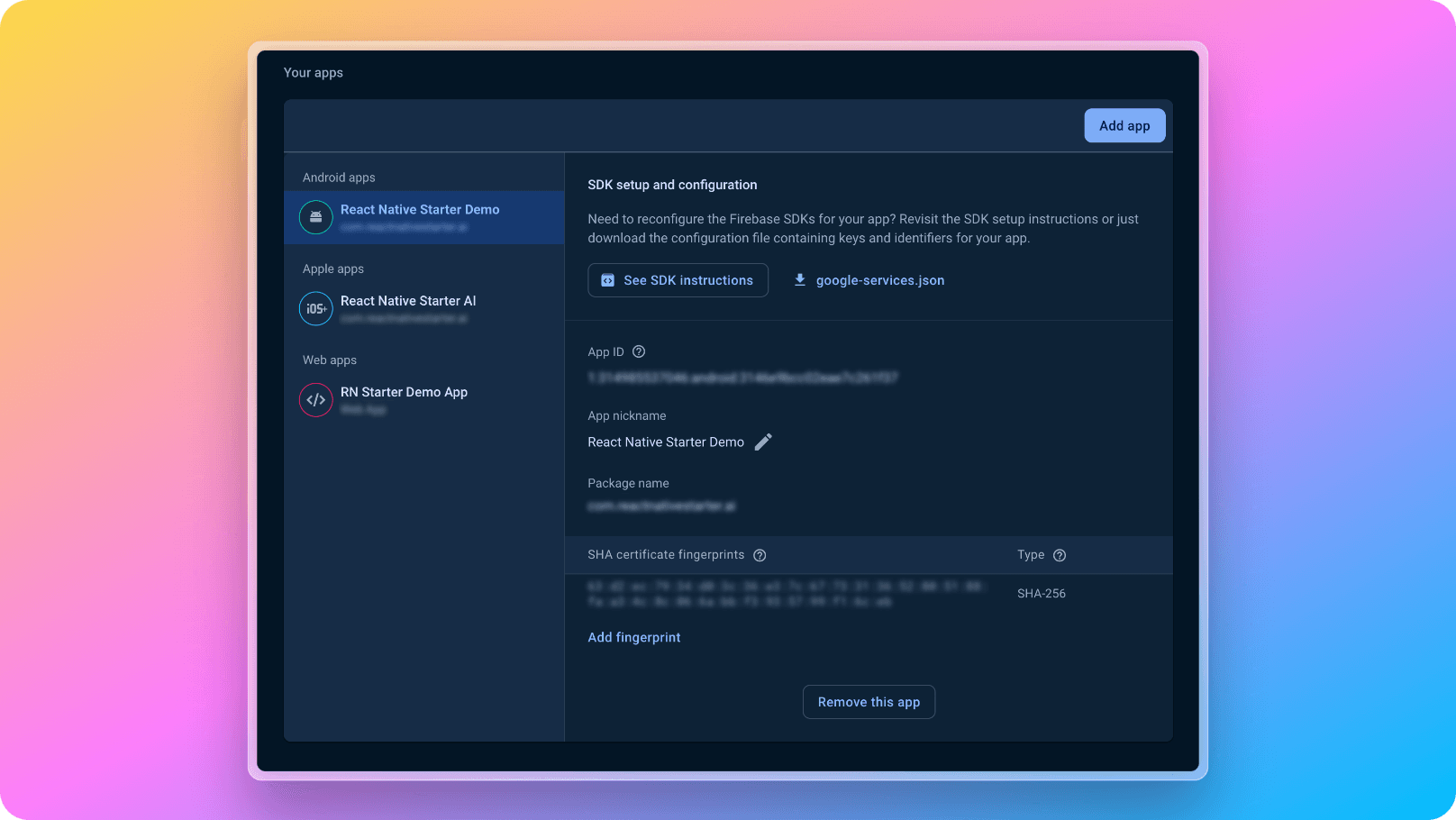
7. Now everything on the codebase side is already built for authenticating your users with Google. You will see the code in react-native-starter-mobile/screens/Authentication/Authentication.tsx page. The Google authentication code is as easy as below:
1 const handleGoogleSignUp = async () => {
2 try {
3 setIsGoogleSignInLoading(true);
4 // Sign-in the user with the credential
5 const user = await onGoogleButtonPress();
6
7 // Update global store with fresh data
8 dispatch(
9 authSlice.actions.setUser({
10 ...(user.user.toJSON() as FirebaseAuthTypes.User),
11 id: user?.user?.uid || "",
12 })
13 );
14 Toast.show({
15 type: "success",
16 text1: "Congratulations!",
17 text2: `You have logged in successfully! 🎉`,
18 });
19 // Save user metadata to NoSQL
20 await saveUserMetadata(user.user.uid);
21 // Go to main tab. You can update this navigation logic depending on your needs
22 navigation.replace("Main");
23 } catch (e) {
24 Toast.show({
25 type: "error",
26 text1: "Error!",
27 text2: `Login failed!`,
28 });
29 console.log("Login error on Google auth");
30 } finally {
31 setIsGoogleSignInLoading(false);
32 }
33 };