Tutorials > In-App Purchases
React Native Starter comes with RevenueCat integration which handles both Google Play Store and Apple App Store payments for you!
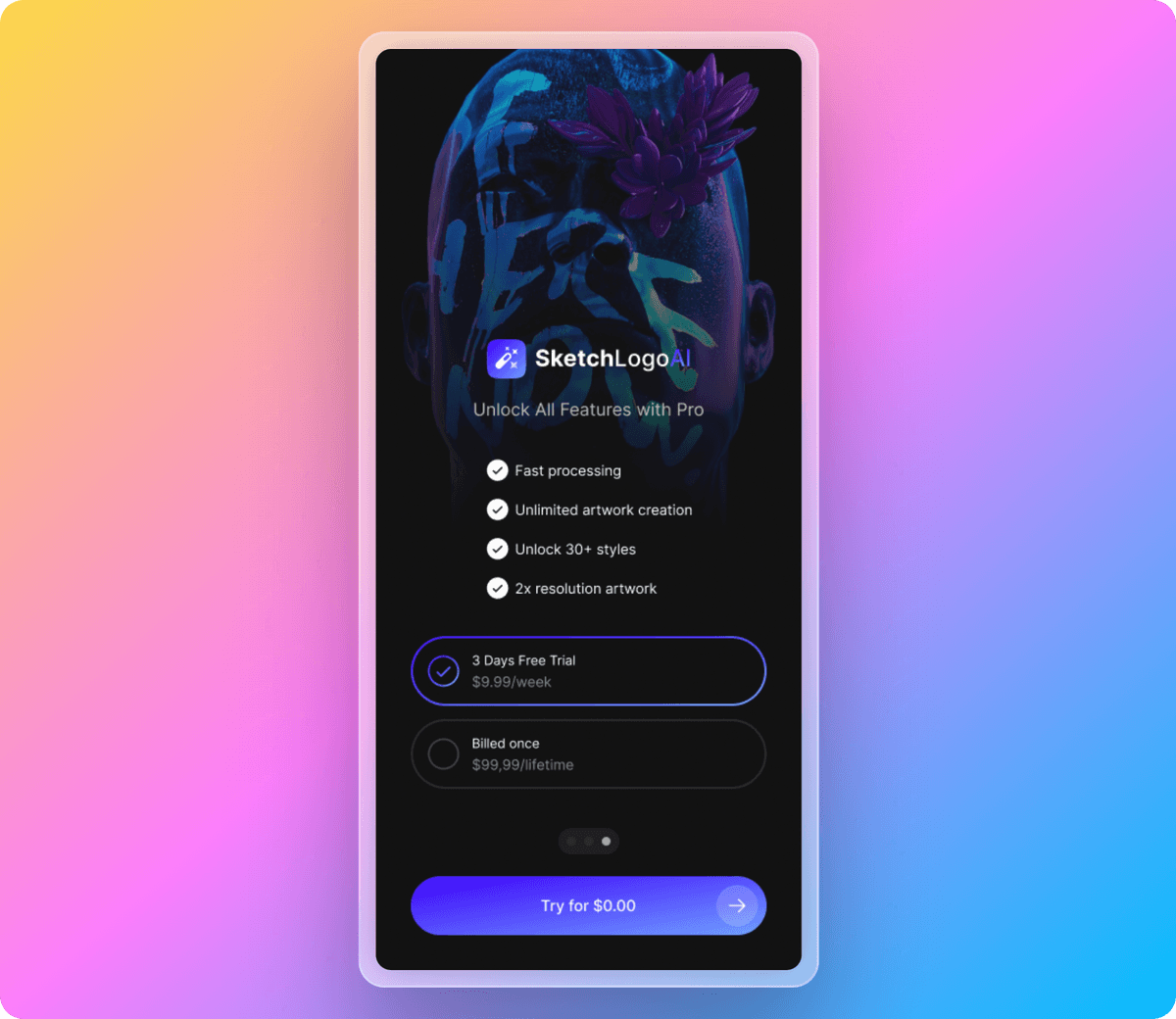
In your app, you may have features that only paying users can access by buying recurring subscriptions. Alternatively, you may need a structure where a certain number of credits are spent per transaction, and these credits are allocated to users when they make a payment.
With React Native Starter, you can securely manage credits and subscriptions using the integration of RevenueCat and Firebase. Thanks to our ready-made paywall screens, all you need to do is define the products and prices.
List the Products on Paywall
1 // Load all offerings a user can (currently) purchase
2 const loadOfferings = async () => {
3 const offerings = await Purchases.getOfferings();
4 if (offerings.current) {
5 setPackages(offerings.current.availablePackages);
6 }
7 if (offerings.all.Credits) {
8 setCreditPackages(offerings.all.Credits.availablePackages);
9 }
10 };
Update the User's State When a Payment Made
1 // Update state when customerInfo loads/changes
2 const updateCustomerInformation = async (customerInfo: CustomerInfo) => {
3 const newUser: UserState = { credits: user.credits, pro: user.pro };
4
5 if (customerInfo?.entitlements.active["Pro"] !== undefined) {
6 newUser.pro = true;
7 setUser(newUser);
8 }
9 };
Listen for Payments on Backend
It is also possible to listen for payments on backend just so that you also can store your users' payments on your database and use it as source of truth.
1export const iapEventHandler = onDocumentCreated(`${IAP_EVENT_COLLECTION}/{id}`, async (event) => {
2 const snapshot = event.data;
3 const data = snapshot.data();
4 const productId = data.product_id;
5 let credits = 0;
6 switch (productId) {
7 case '25_credits':
8 credits = 25;
9 break;
10 case '50_credits':
11 credits = 50;
12 break;
13 case '100_credits':
14 credits = 100;
15 break;
16 }
17
18 if (credits <= 0) {
19 console.log(`No credits to give for productId(${productId})`);
20 return;
21 }
22 const db = getFirestore();
23 const userRef = db.collection(USER_COLLECTION).doc(data.app_user_id);
24
25 // Add credits to user
26 await userRef.update({
27 credit: FieldValue.increment(credits),
28 });
29
30 });
In the above example, when one of the three different non-consumable products with options of 25, 50 and 100 credits is purchased, a Firebase serverless function is triggered to increase the credit count of the respective user in the database.