ESC
Tutorials > Private Screen
🔐 You might need some pages that you only want your logged in users to be able to see such as profile/account page etc. It is pretty easy to configure your protected app screens with React Native Starter AI!
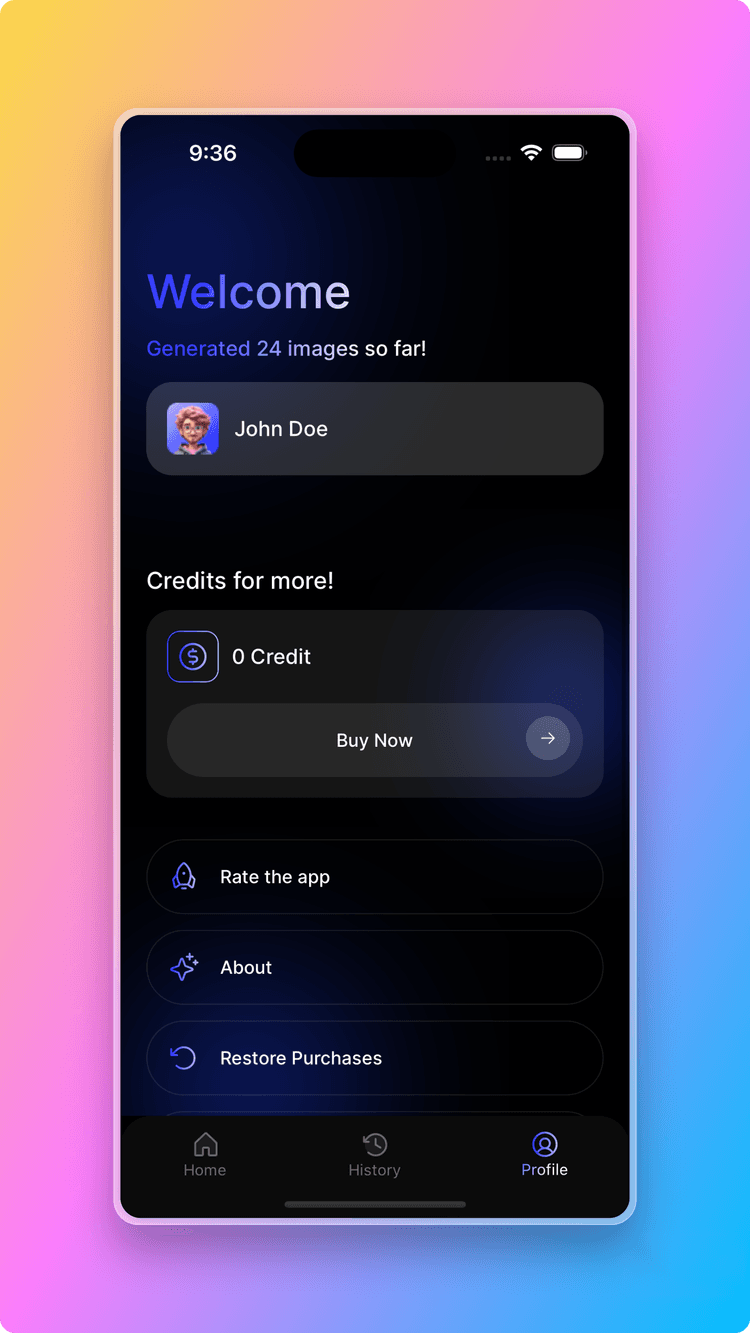
Protected API
🔒 Need to have protected API calls? 🚀 Follow the Protected API tutorial to create new ones easily!🔒 We already have a authentication store in the codebase. Every time a user logs in or logs out, the store's values are getting updated and the store is getting populated by the user information.
And the authentication store is also persistent; so across closing and opening the mobile application, your users stay authenticated because it uses React Native Async Storage under the hood along with the store logic.
Check this out! A simple profile screen showing the user's data:
react-native-starter-mobile/screens/tabs/Profile/Profile.tsx
1import React, { useEffect } from "react";
2 import {
3 View,
4 StyleSheet,
5 Text,
6 TouchableOpacity,
7 Image,
8 ScrollView,
9 } from "react-native";
10 import auth from "@react-native-firebase/auth";
11
12 import { useSafeAreaInsets } from "react-native-safe-area-context";
13 import { NativeStackNavigationProp } from "@react-navigation/native-stack";
14 import { useNavigation } from "@react-navigation/native";
15 import { RootStackParamList } from "../../index";
16 import MaskedView from "@react-native-masked-view/masked-view";
17 import { LinearGradient as ExpoLinerGradient } from "expo-linear-gradient";
18 import Svg, { Defs, LinearGradient, Path, Rect, Stop } from "react-native-svg";
19 import { useAppDispatch, useAppSelector } from "store";
20 import { authSlice } from "store/slices/auth.slice";
21
22 const ProfileScreen = () => {
23 const dispatch = useAppDispatch();
24 const userUid = useAppSelector((state) => state.auth.user?.uid);
25 const userFullname = useAppSelector((state) => state.auth.user.name);
26 const userProfilePhoto = useAppSelector((state) => state.auth.user.profilePicture)
27
28 // Here we are checking the authentication status and redirecting the user if not authenticated
29 useEffect(() => {
30 if (!userUid) {
31 navigation.replace("Authentication");
32 }
33 }, [userUid]);
34
35 const handleSignOut = async () => {
36 await auth().signOut();
37 dispatch(authSlice.actions.setUser(null));
38 };
39
40 return (
41 <ScrollView
42 contentContainerStyle={{ ...styles.container, marginTop: insets.top }}
43 >
44 <View style={styles.avatarContainer}>
45 <Image
46 source={{uri: }}
47 style={{ width: 40, height: 40 }}
48 />
49 <Text style={styles.nameText}>{user.name}</Text>
50 </View>
51
52 <View>
53 <View style={{ marginTop: 32 }}>
54 <TouchableOpacity
55 onPress={handleSignOut}
56 >
57 <Text style={styles.bottomButtonText}>Sign Out</Text>
58 </TouchableOpacity>
59 <View style={{ height: 70 }} />
60 </View>
61 </View>
62 </ScrollView>
63 );
64 };
65
66 const styles = StyleSheet.create({
67 container: {
68 padding: 20,
69 position: "relative",
70 },
71 avatarContainer: {
72 marginTop: 16,
73 backgroundColor: "rgba(255, 255, 255, 0.16)",
74 borderRadius: 20,
75 padding: 16,
76 flexDirection: "row",
77 alignItems: "center",
78 gap: 12,
79 },
80 bottomButtonText: {
81 color: "white",
82 fontFamily: "Inter-Medium",
83 fontSize: 14,
84 },
85 });
86
87 export default ProfileScreen;
88