Tutorials > User Authentication
React Native Starter comes with an Authentication Screen that includes Email/Password, Google and Apple authentication out of the box for you!
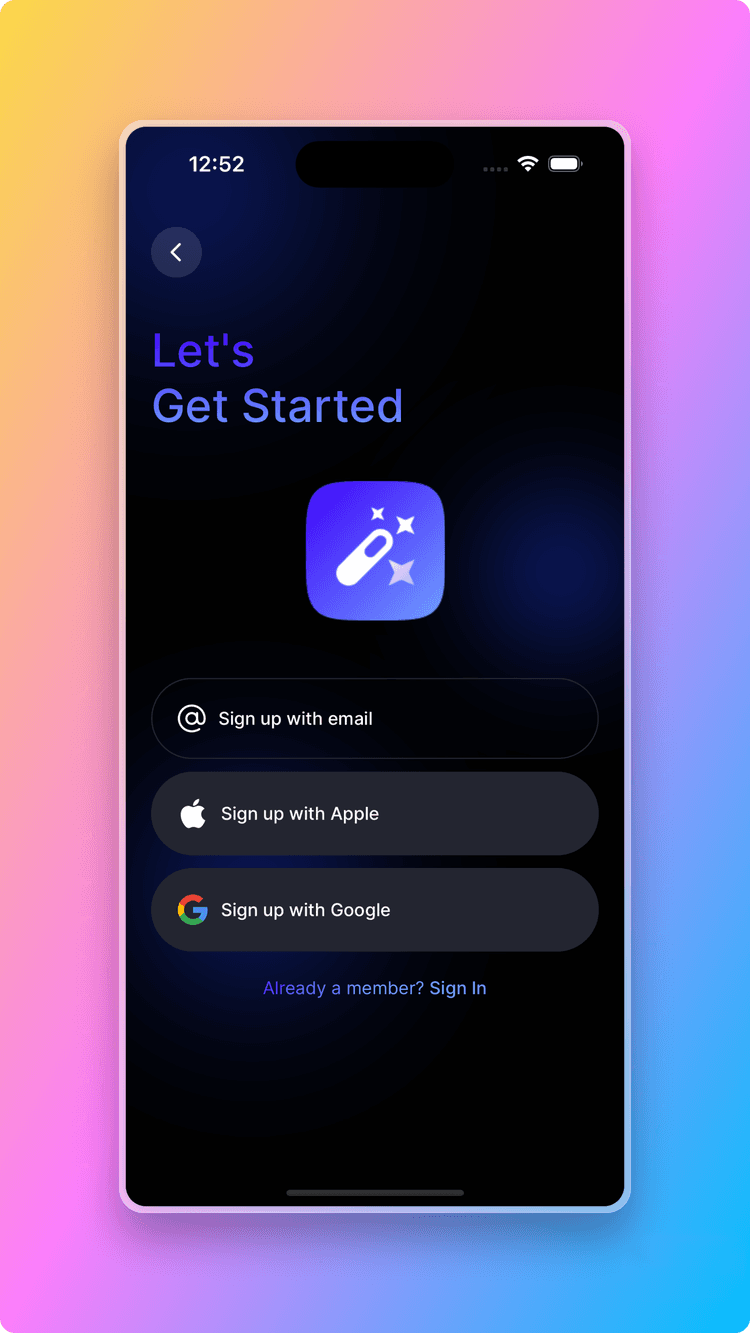
It is pretty easy to move this authentication logic into any place that you wish or you can directly setup your mobile app's authentication by using the 2 sample social authentication handler functions below.
Google Authentication
1import { onGoogleButtonPress } from "utils/on-google-button-press";
2
3// Sign-in the user with the credential
4const user = await onGoogleButtonPress();
5
6// Update global store with fresh data
7dispatch(
8 authSlice.actions.setUser({
9 ...(user.user.toJSON() as FirebaseAuthTypes.User),
10 id: user?.user?.uid || "",
11 })
12);
13Toast.show({
14 type: "success",
15 text1: "Congratulations!",
16 text2: 'You have logged in successfully! 🎉',
17});
18// Go to main tab. You can update this navigation logic depending on your needs
19navigation.replace("Main");
Apple Authentication
1import { onAppleButtonPress } from "utils/on-apple-button-press";
2
3// Sign-in the user with the credential
4const user = await onAppleButtonPress();
5if (user) {
6 // Update global store with fresh data
7 dispatch(
8 authSlice.actions.setUser({
9 ...(user.user.toJSON() as FirebaseAuthTypes.User),
10 id: user?.user?.uid || "",
11 })
12 );
13 Toast.show({
14 type: "success",
15 text1: "Congratulations!",
16 text2: 'You have logged in successfully! 🎉',
17 });
18 // Go to main tab. You can update this navigation logic depending on your needs
19 navigation.replace("Main");
20}
If you need to use Email/Password authentication in your mobile application, React Native Starter AI already comes with a UI built with functionality as below. It is easy and fast to embrace it in your application!
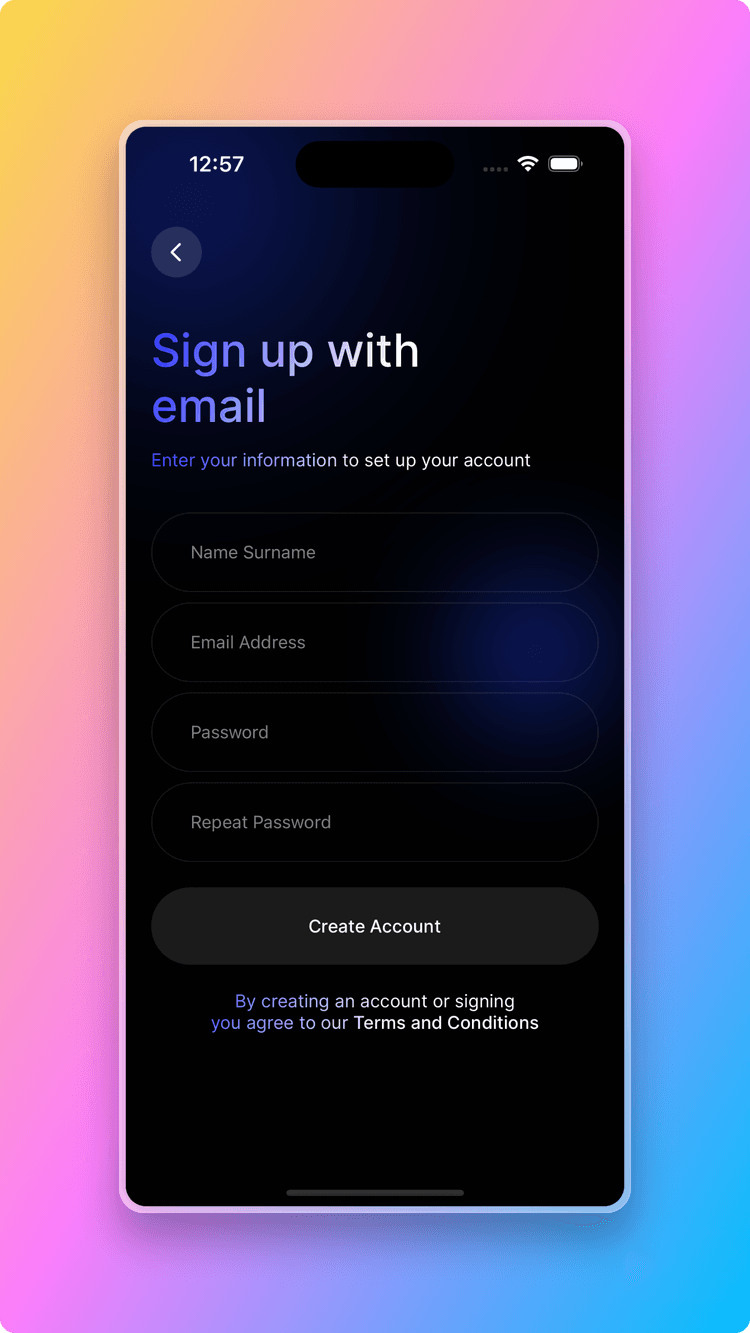
It is also possible to authenticate your users anonymously just so that you don't need your users' email address or any other information and just want to associate them to an in-app purchase without degrading the user experience of getting their personal info depending on your needs in your mobile app.
Anonymous Authentication
1// Sign-in the user with the credential
2const user = await auth().signInAnonymously();